How to Fix Missing Meshes in a Three.js Scene
- Published on
- Authors
- Name
- Jerry
Recently, I encountered a small issue while using react-three-fiber. After using the command-line tool gltfjsx to convert a glTF file into a React component and adding this component to the scene, certain meshes would disappear when the camera moved to specific positions.
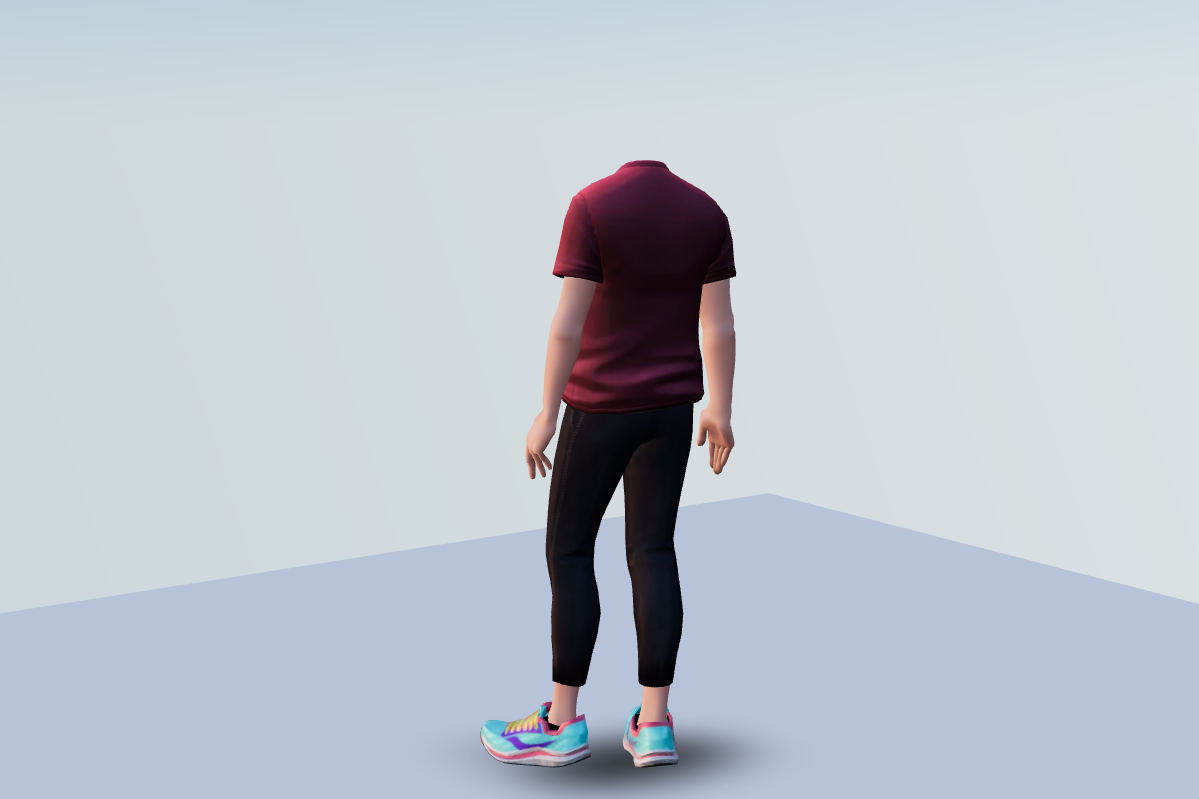
“Ah! The head is gone!”
After some investigation, I discovered that this was caused by the frustumCulled property, which is set to true by default. According to the three.js documentation:
When this is set, it checks every frame if the object is in the frustum of the camera before rendering the object. If set to
false
the object gets rendered every frame even if it is not in the frustum of the camera. Default istrue
.
The fix for this issue is quite simple: just set the frustumCulled property of the relevant mesh to false.
// ...
return (
<group {...props} dispose={null}>
<primitive object={nodes.Hips} />
<skinnedMesh
geometry={nodes.Wolf3D_Hair.geometry}
material={materials.Wolf3D_Hair}
skeleton={nodes.Wolf3D_Hair.skeleton}
frustumCulled={false}
/>
// ...
<skinnedMesh
name="Wolf3D_Head"
geometry={nodes.Wolf3D_Head.geometry}
material={materials.Wolf3D_Skin}
skeleton={nodes.Wolf3D_Head.skeleton}
frustumCulled={false}
morphTargetDictionary={nodes.Wolf3D_Head.morphTargetDictionary}
morphTargetInfluences={nodes.Wolf3D_Head.morphTargetInfluences}
/>
<skinnedMesh
name="Wolf3D_Teeth"
geometry={nodes.Wolf3D_Teeth.geometry}
material={materials.Wolf3D_Teeth}
skeleton={nodes.Wolf3D_Teeth.skeleton}
frustumCulled={false}
morphTargetDictionary={nodes.Wolf3D_Teeth.morphTargetDictionary}
morphTargetInfluences={nodes.Wolf3D_Teeth.morphTargetInfluences}
/>
</group>
)
// ...
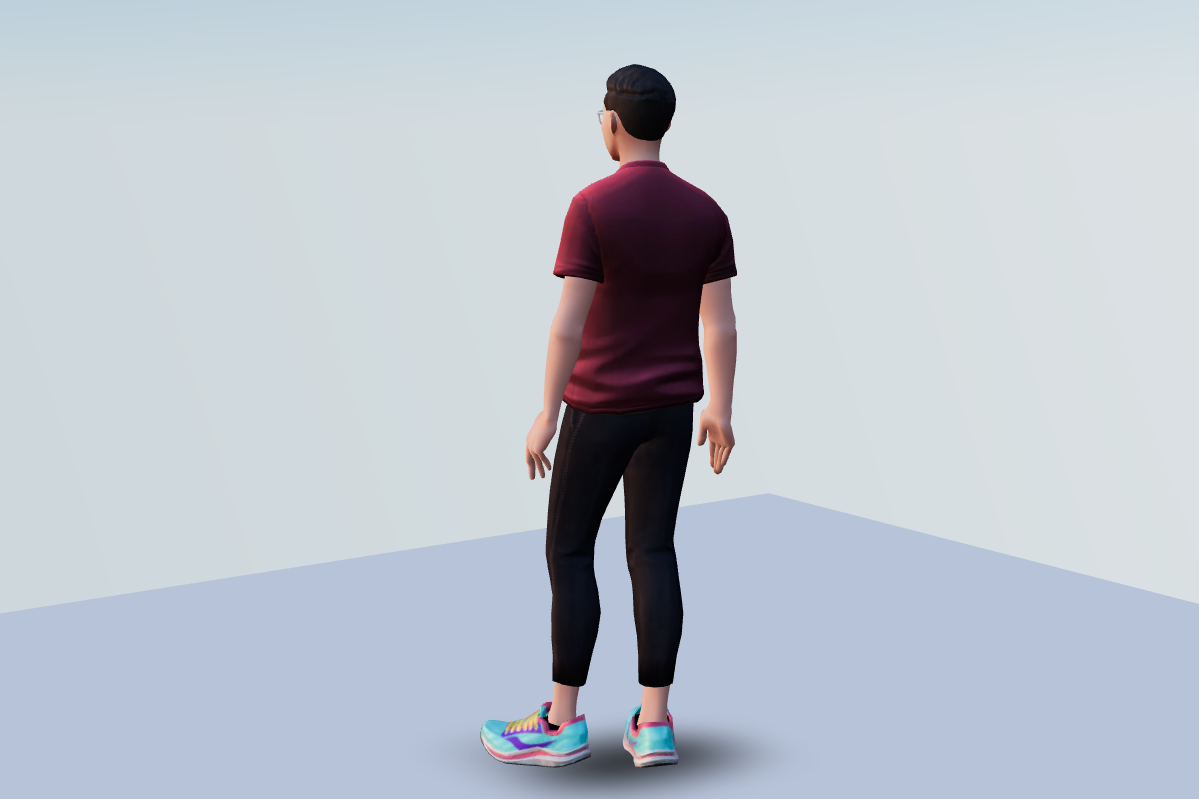
“The head is back now!”