How to Perform Different Actions When a Web Page Is Embedded/Not Embedded in a Mobile App
- Published on
- Authors
- Name
- Jerry
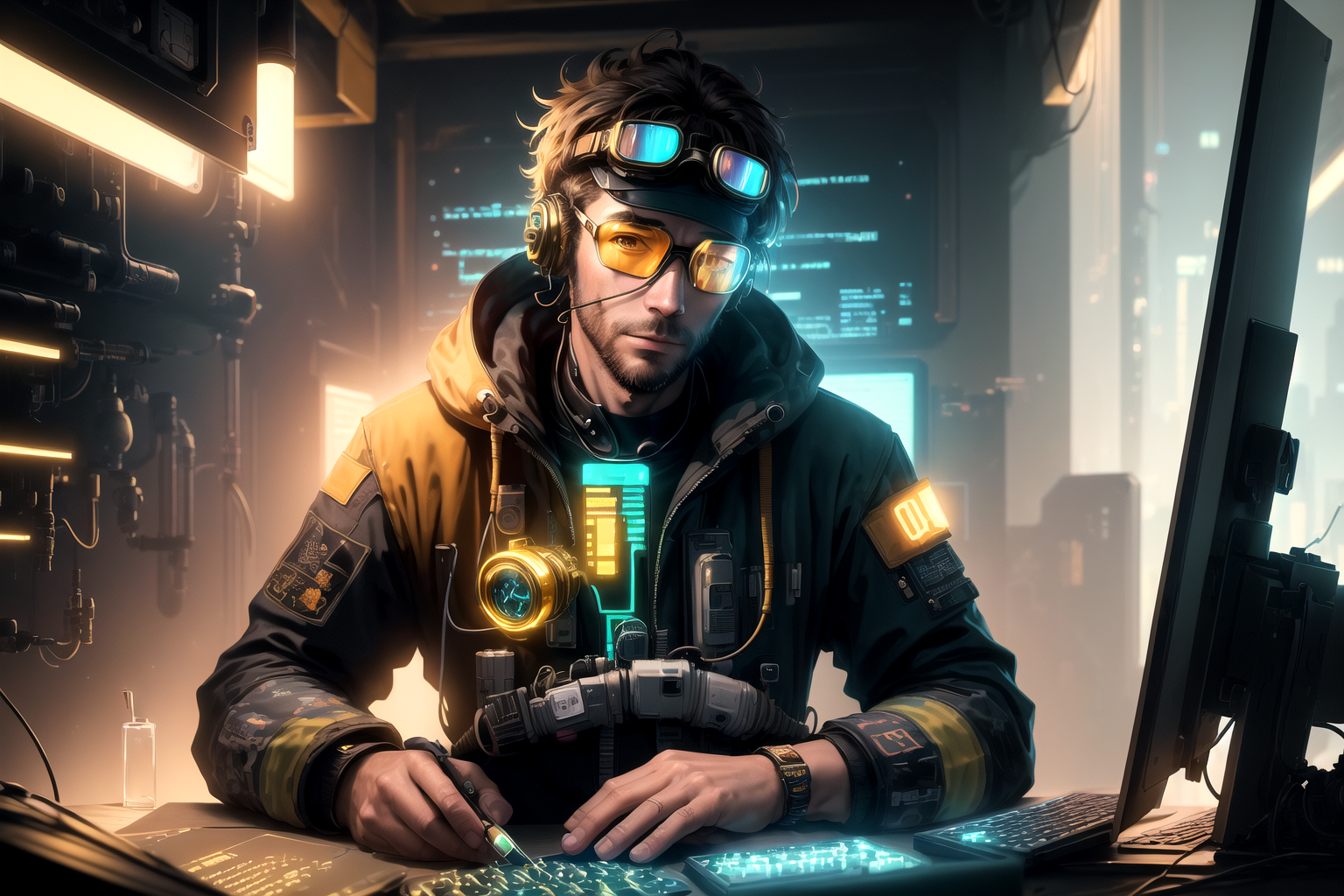
(Image generated by Stable Diffusion WebUI)
While the use cases may be limited, there are times when we indeed need to execute different behaviors when a web page is embedded. For example, when a web page is embedded, we may need to hide certain elements, or we may need to perform specific actions when the page is embedded.
In my work last month, I encountered such a requirement: I needed to create a page where one button triggers a functionality within the native iOS/Android app when the page is embedded, and when the page is opened in a browser, the button triggers the page's own logic.
Upon receiving the task, I communicated with my iOS/Android colleagues. They informed me that when they embed the web page using a webview, they define functions for the page that can be called by the web page, thereby triggering the functionality of the native app. They provided me with the functions' name, and all I had to do was bind the click event of the button in the web page to these iOS/Android functions to invoke the native app's functionality.
However, I realized that I also needed to consider a logic: when the web page is opened in a browser, these functions do not exist. If I directly call them in the web page, errors will occur, which is obviously not the desired result. Additionally, I needed to handle the scenario where the page is opened in a browser, and the button's click event should be bound to the page's own logic.
Initially, I considered using the userAgent to determine whether the web page is opened in a browser or embedded in a native app. However, I quickly found that this method is unreliable because the userAgent in webview can be the same to that in the browser, and userAgent varies across different browsers. Therefore, this method is not dependable.
Later, I attempted to implement the functionality of the native app first. I directly bound the function names provided by iOS/Android colleagues to the click event of the button, and they worked well in the native apps. However, when I opened the web page in a browser, as expected, an error occurred because this function did not exist.
So, I thought of using a try-catch statement to catch this error. If the error is caught, it indicates that the function does not exist, and I can then execute the web page's own logic.
function handleButtonClick() {
// Assume the environment is in the app
let isInIosApp = true
let isInAndroidApp = true
// Attempt to call the Android app's method; if it fails, it means it's not within the app
try {
AndroidFunction.reserveFunction(message)
} catch (androidError) {
isInAndroidApp = false
}
// Attempt to call the iOS app's method; if it fails, it means it's not within the app
try {
window.webkit.messageHandlers.reserveFunction.postMessage(message)
} catch (iosError) {
isInIosApp = false
}
if (!isInAndroidApp && !isInIosApp) {
// If not within either app, execute the web page's own logic
// ...
}
}
In this development, I utilized a try-catch statement, which is not commonly used, to capture the error of the non-existent function. This way, I could perform different actions when the web page is embedded.